티스토리 뷰
포스팅에 앞서 내용이 틀릴 수 있습니다.
해당 부분 지적 감사히 받습니다.
자바를 코딩해 본 사람이라면 무조건 접해봤을 오류다.
바로 NullPointerException이다.
이것이 왜 발생하는지 먼저 알아보자
말 그대로 Null + Pointer 널 포인터 예외이다.
근데 포인터는 c 언어에서 나오는 것 아닌가?
맞지만 아니다.
자바에서도 Pointer가 있다.
바로.이다.
아래 예시를 보자
public class Student{
String name;
int age;
}
public class test1 {
public static void main(String[] args) {
Student st = new Student();
System.out.println(st.name);
}
}
여기선 어디가 포인터 일까?
바로
st.name
이 부분의. 이 Pointer이다.
자바에서 객체는 주솟값을 가진다고 이야기했었다.
이 주솟값이 비어있기 때문에 NullPointerException이 발생하는 것이다.
사실 이것만 알아도 앞으로 발생할 NullPointerException에 대해 모두 대비할 수 있다.
일단 한번 발생시켜 보자.
public class test1 {
public static void main(String[] args) {
Student st = null;
System.out.println(st.name);
}
}
실행 결과
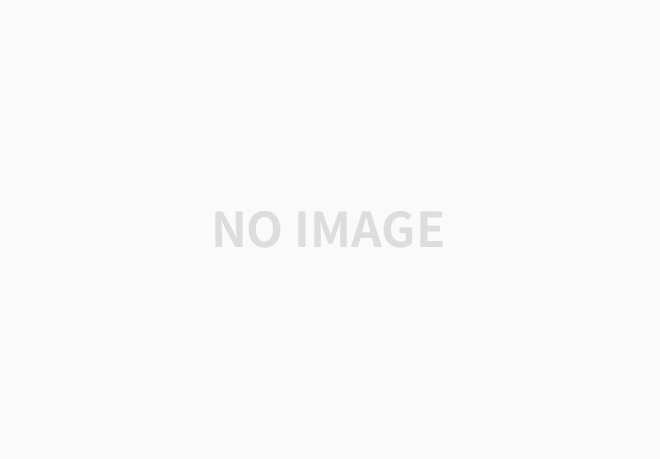
짜잔 NullPointerException이 발생하였다.
NullPointerException 이 발생하면 코드는 해당 라인에서 수행이 중단되며 프로그램이 종료된다.
물론 예외처리를 하면 프로그램이 진행되게 끔 유지할 수 있다.
조금 더 자세히 알아보자
NullPointerException는 참조할 주솟값이 비어있을 때 (Null) 발생한다.
이것을 잘 기억해 두자.
한 번만 더 꼬아보자
public class Data {
int value;
}
public class Student{
String name;
int age;
Data data;
}
위와 같은 Class를 생성하였다.
아래 코드 실행 예시는 어떻게 될까?
public class test1 {
public static void main(String[] args) {
Student st = new Student();
System.out.println(st.data.value);
}
}


바로 NullPointerException이 발생한 것을 확인할 수 있다.
st.data.value
이 부분에서 문제가 발생한 것인데, st까지는 괜찮았지만,. data에서 주솟값이 Null로 들어가 있기 때문이다.
Null인 이유는. 지역 변수의 경우 사용자가 지정할 때 반드시 초기 값을 설정해 주어야 오류가 발생하지 않는다.
Class 즉 인스턴스 변수를 생성할 때는 초기 값을 설정해 주지 않아도 오류가 발생하지 않는데, 자바가 초기값을 자동으로 설정해 주기 때문이다.
기본형 은 0으로, 참조형은 Null로 초기값을 세팅해 준다.
따라서 Student 객체의 Data 부분에는 초기값이 없어 Null로 세팅되어 있을 것이고, 이 부분에서 NullPointerException이 발생한 것을 확인할 수 있다.
그러면 이것을 단순히 해결해 보자.
public class test1 {
public static void main(String[] args) {
Student st = new Student();
st.data = new Data();
System.out.println(st.data.value);
}
}
st.data = new Data(); 를 추가하였다
결과를 보자
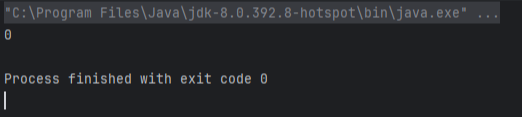
아까말한 대로 자바가 초기화해주는 Class 변수 기본형의 값으로 0이 들어가 있는 것을 확인할 수 있다.
추가적으로 단순히 참조값만 준다고 아래와 같이 하면 오류가 발생한다.
public class test1 {
public static void main(String[] args) {
Student st = new Student();
st.data = new Student();
System.out.println(st.data.value);
}
}
왜냐하면.. Student Class의 data의 타입이 data이기 때문에 타입이 달라 오류가 발생한다.
자바에서는 같은 타입의 객체만 할당할 수 있다.
이 부분 또한 명심해 두자.
String에 int 형을 넣을 수 없듯이..
또한 추가로 Student와 Data의 객체의 형태를 똑같이 만들어 보았다.
public class Student{
String name;
int age;
Data data;
}
public class Data {
String name;
int age;
Data data;
}
그럼에도 불구하고
public class test1 {
public static void main(String[] args) {
Student st = new Student();
st.data = new Student();
}
}
st.data = new Student(); 부분에서 오류가 난다.
Class의 형태가 같아도 다른 Class 이기에 자바에서는 다른 객체로 인식한다.
따라서 위 코드는 불가능하고
아래 코드가 맞다
public class test1 {
public static void main(String[] args) {
Student st = new Student();
st.data = new Data();
}
}
'기술스택 > 자바(Spring)' 카테고리의 다른 글
자바 메모리 구조 - 메서드, 스택, 힙 영역 (2) | 2025.02.08 |
---|---|
자바 접근제한자(Access Modifier) + 캡슐화(Encapsulation) (1) | 2025.02.07 |
자바 기본 형/참조 형 (+Garbage Collection) (0) | 2025.02.05 |
자바 클래스(static)와 인스턴스(non-static) 접근 가능 방향 (1) | 2025.02.05 |
자바 Scanner의 고질병 (2) | 2025.02.04 |
- Total
- Today
- Yesterday
- Los
- 김영한 실전 자바 기초
- 프로그래머스
- 김영한 실전 자바 중급
- webhacking.kr
- lord of sql
- 기술스택
- 상속
- extends
- 백준 피보나치 수열
- zixem
- los 15
- 코딩테스트 준비
- 백준 피보나치
- samron3
- 프로그래머스 상품을 구매한 회원 비율 구하기
- 김영한 실전 자바 기본
- 코딩테스트
- spring
- 자바
- ys.k
- 김영한
- samron
- los 15단계
- 상품을 구매한 회원 비율 구하기 파이썬
- 백준
- static
- 프로그래머스 상품을 구매한 회원 비율 구하기 파이썬
- 스프링
- java
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | ||||
4 | 5 | 6 | 7 | 8 | 9 | 10 |
11 | 12 | 13 | 14 | 15 | 16 | 17 |
18 | 19 | 20 | 21 | 22 | 23 | 24 |
25 | 26 | 27 | 28 | 29 | 30 | 31 |